I’m currently working on a PHP web application and would like to skip the login page by automatically authenticating a user using his Windows logon credentials. Quite simply I just wanted to get which user is currently logged in the system, and use that variable to query the database and display relevant information on the web page.
By getting the authenticated username value, we eliminate the need for a login form because the user has already been authenticated the moment he logs into his computer.
Ideally, intranet applications should be using single sign on to avoid redundancy in forms. But LDAP authentication should still be present to handle certain scenarios that still need login screens. So here’s how to implement it if you’re running a PHP web application served using IIS manager.
What you need to do is enable Windows Authentication on IIS manager and use $_SERVER[‘AUTH_USER’] on PHP to retrieve the windows logged-in user:
If you don’t have Windows Authentication installed on IIS, here’s how.
- Open Server Manager (Start > All Programs > Administrative Tools > Server Manager)
- Navigate to Roles > Web Server (IIS)
- Right-click on Web Server (IIS) and select Add Role Services.
- Scroll down on the list of services and find Windows Authentication under Security.
- Select and install.
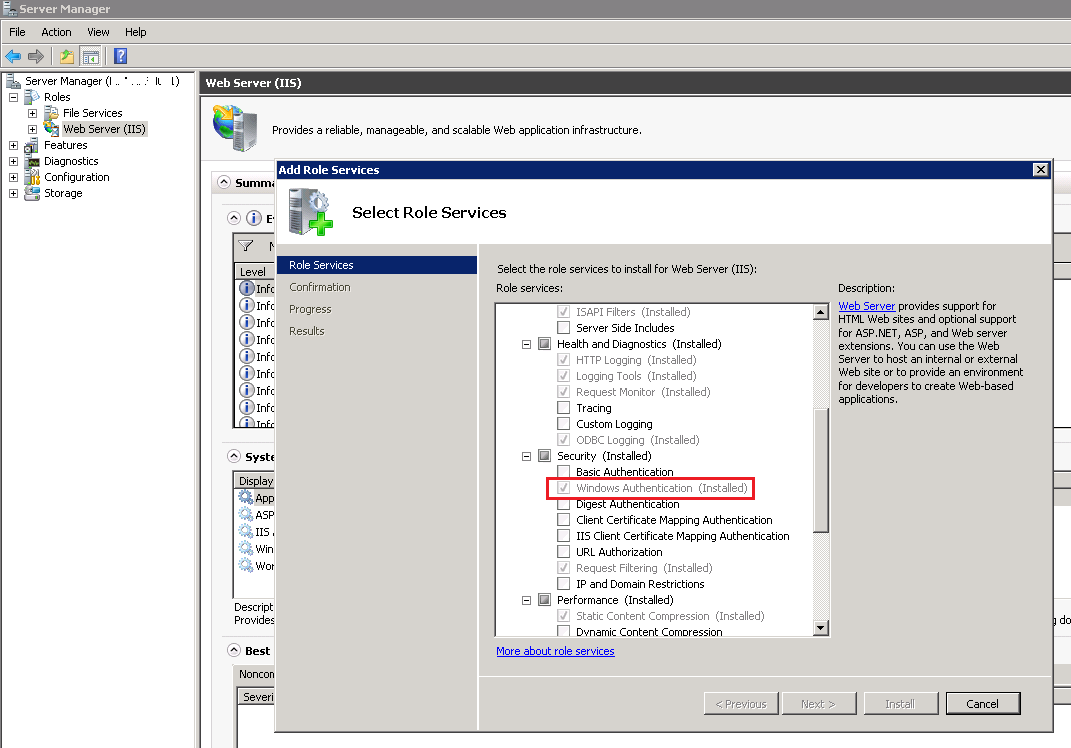
Once Windows Authentication is installed, go to IIS Manager, navigate to your site and enable it under IIS > Authentication. Also make sure to disable Anonymous Authentication to avoid conflict.
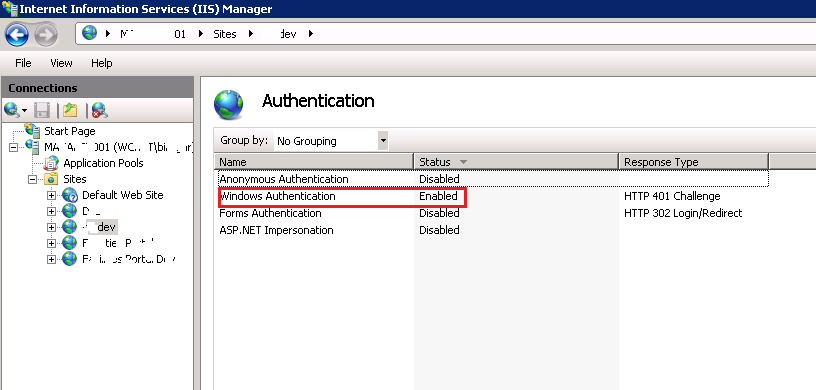
Now to back to your PHP code, as mentioned, $_SERVER[‘AUTH_USER’]returns the domain and username of the currently logged in person, ie: DOMAIN\myusername01
If you want to extract just the username, you can use PHP’s str_replace() function, ie:
str_replace("DOMAIN\\","",$_SERVER['AUTH_USER']);
This returns the naked username, which you can then use to query your database for validation.
That’s it, hope this helps! 🙂